Here’s a quick cheat sheet for lots of design patterns. The examples in thispost are linked to Java examples however they are not limited to a singlelanguage implementation. Pubg mac os x. Special thanks to Derek Banas.
- Software Architecture Patterns Cheat Sheet
- Stock Chart Patterns Cheat Sheet
- Software Patterns Cheat Sheet Free
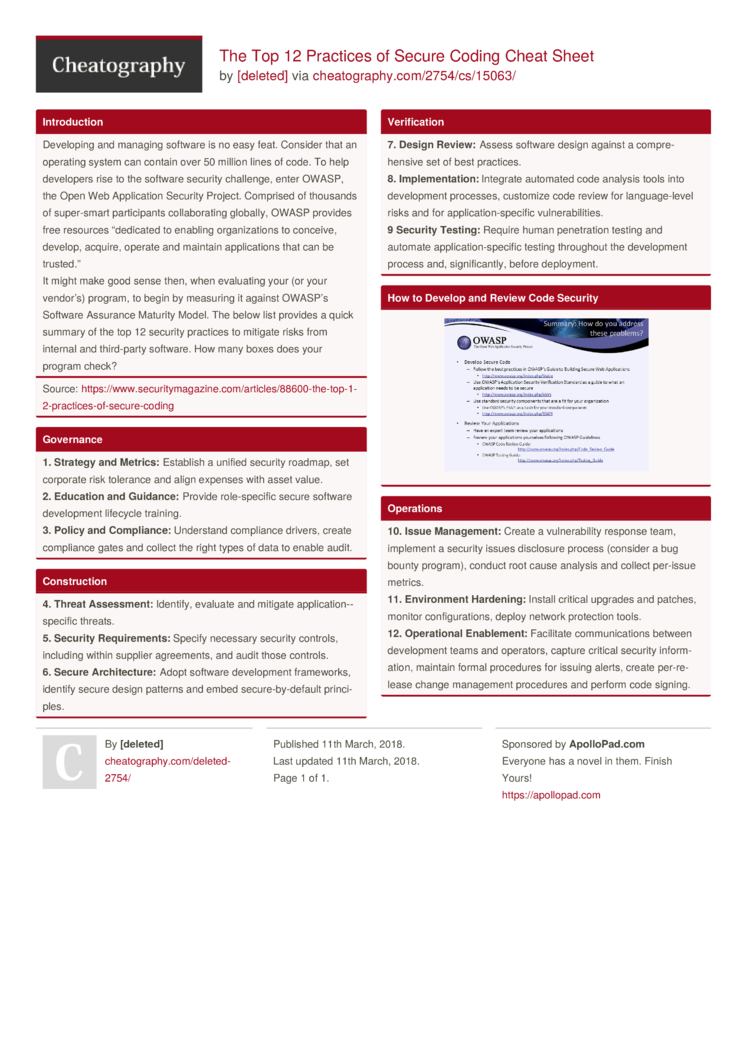
- Software design patterns cheatsheet. Contribute to SuperV1234/patterns-cheatsheet development by creating an account on GitHub.
- Java Design Pattern Cheat Sheet. Saved by allwynv. Computer Coding Computer Programming Computer Science Software Design Patterns Pattern Design Object Oriented Design Patterns Design Patterns In Java Web Design Diagramas Uml.

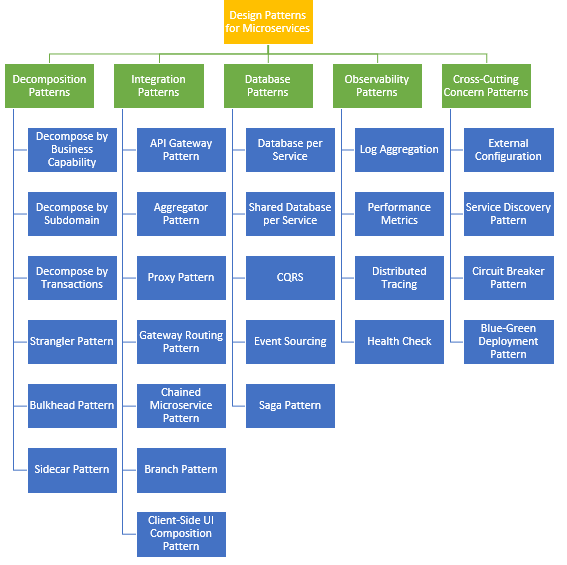
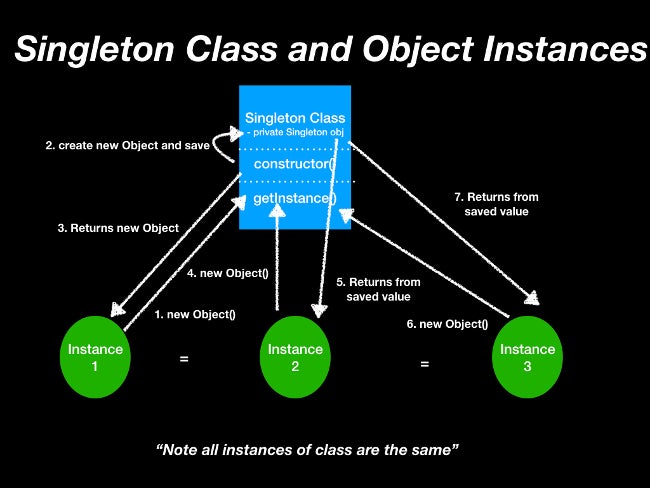

Software Architecture Patterns Cheat Sheet
- Observer Design Pattern: Contains an object usually referred to asthe
subject
that maintains a a list of dependent classes calledobservers
.Thesubject
object notifies allobservers
automatically of any state changesby calling their methods. - Factory Design Pattern: Creates objects that share the same superclass type. Usually has a method named like
makeTypeOfSuperClass
which acceptsan argument to tell it what to do. - Abstract Factory Design Pattern: Groups together individualfactories. The objects that the these factories return share a common API.
- Singleton Design Pattern: Used to eliminate the option of instantiatingmore than one object. Contains a static variable that holds the first instanceof the object created. This object is always is returned. Typically returned byusing a
getInstance
method. The constructor is set as a private function toprevent users instantiating it. - Builder Design Pattern: Builder objects contain methods that tell afactory how to build/configure an object.
- Prototype Design Pattern: Creates new objects by copying otherobjects. Nice to use a clone factory that accepts an object to clone.
- Decorator Design Pattern: Adds functionality by using many simpleclasses.
- Command Design Pattern: Allows you to set aside a list of commandsfor later use. Each command is typically it’s own command object that implementsthe same command interface.
- Adapter Design Pattern: Allows 2 incompatible objects that share asimilar purpose to work transparently by abstraction.
- Facade Design Pattern: Decouples or separates the client from all ofthe sub components. Main purpose is to simplify interfaces so you don’t have toworry about what’s going on under the hood. Similar to a
Service Design Pattern
except a Service typically communicates on external service. - Bridge Design Pattern: Defines 2 layers of abstraction. One for thetarget which can be extended for different types of receivers and one for thecontrols which can be extended to different type of controls that will be ableto communicate with the targets.
- Template Design Pattern: Contains a usually a final method thatdefines the steps of an algorithm. It allows subclasses to configure thetemplate by overwriting methods.
- Iterator Design Pattern: Provides a uniform way to access differentcollection types of objects. For instance, creating an iterator interface thatyour collections of Arrays, Lists, or Maps can implement so you can iterate overthem the same way.
- Composite Design Pattern: Allows you to attach individual objectsand a composition of objects uniformly. For example, imagine a folder treestructure starting at root. This can be the root composite object (aka folder)where it accepts types of files and types of folders. File types have no childcomponents attached to them but a folder can have many more files and even moregroupings contained within.
- Flyweight Design Pattern: Used when creating a lot (i.e.: 100,000 ormore) of similar objects. Objects will be created from a factory that checks ifa pre-existing object does not exist that shares a similar definition. If a pre-existing object exists with a similar configuration then the factory willreturn this rather creating a new object and reconfiguring.
- State Design Pattern: Allows an object to change it’s behavior whenit’s state changes. Each state implements a behavior associated with it’s stateof context. Should be implemented with as few states as possible. Ie: Imagine adatabase connection that has two states (connected and disconnected) and aquery method. The connection object execute the query method differentlydepending on it’s connection state.
- Proxy Design Pattern: Provides a class with limited access toanother class. This is done for security reasons.
- Chain of Responsibility Design Pattern: Sends problem to an objectand if that object can’t use it, then it sends it to an object chained to itthat might. The object chained to it can have an additional object chained andwill continue to run it’s course until it finds an object that can solve theproblem.
- Interpreter Design Pattern: This pattern solves a problem by using acontext object to interpret an issue and find a class using reflection to returnthe answer.
- Mediator Design Pattern: Handles communication between relatedobjects without having to know anything about each other. Objects are typicallyconstructed with the mediator passed in. Mediator will regulate interactions.
- Memento Design Pattern: Useful for saving history states of anobject. The object stored in state is referred to as the Memento. Mementos aremanaged by caretakers contain a list of each version for retrieval. Lastly, wehave an originator which is responsible for communicating with the caretaker tosave and restore states. Imagine a save, under, and redo scenario.
- Visitor Design Pattern: Allows you to perform the same action onmany objects of different types without much alteration to those classes. Aconcrete Visitor class will implement a common visit method for each type ofvisitor. Each visitable will implement a Visitable interface that implements anaccept method. Upon usage, each visitable object can pass a type of visitorobject to perform different functions. Extremely useful for creating new ways ofVisitors without touching Visitable classes.
State machine:-It used to describe various states of a single component throughout the software development life cycle. Their are 4 type of state in state machine:- Initial state:-The initial state symbol is used to indicate the beginning of a state machine diagram. Watch simmba hd movie online. Final state:- This symbol is used to indicate the end of a state machine.
Stock Chart Patterns Cheat Sheet
Further Reading
Software Patterns Cheat Sheet Free
- JavaScript Design Patterns: A free book by O’Reily showing many ofthe above design patterns implemented in JavaScript.
- DesignPatternsPHP Projects: Great code examples of implementing theabove design patterns (and more) in PHP.
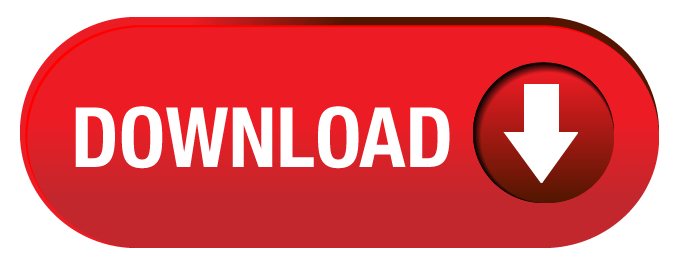